WordPress has the Block Styles API in version 5.3 as a feature for theme authors to create block customizations.
In this post, we will learn about block styles, when to use them, and how to build them from scratch.
What is block style?
A block style is nothing more than a CSS class and, this class can be attached to a block’s output to change its design. Theme authors can add any CSS properties and values to it.
We can register any number of styles for individual blocks, and WordPress will display them in the block’s sidebar panel in the editor. Users can select their desired style shown in the following screenshot:
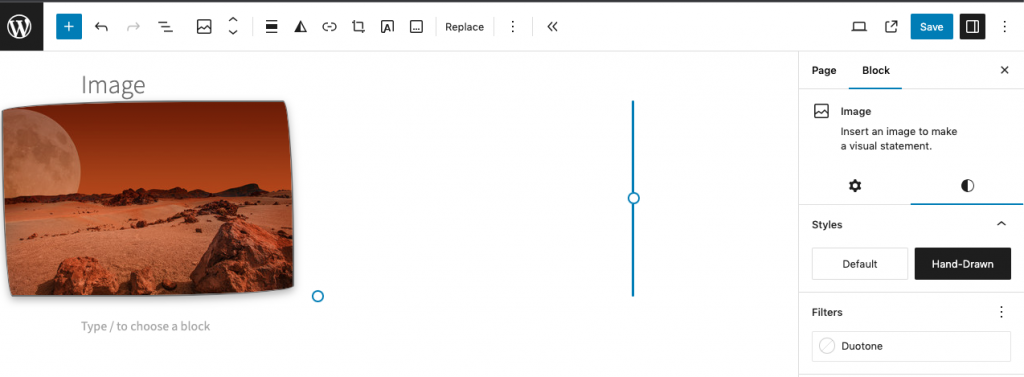
Time to make a custom block style?
You should use block styles when you need to rely on custom CSS. If you want to give an Image block a solid border, there is no need for a custom style as WordPress already has built-in options.
How to Register a custom block style?
The first step is to use the register_block_style()
PHP function.
register_block_style(
$block_name,
$style_properties
);
The $block_name
parameter is the name of the block type (e.g., core/image
). The $style_properties
parameter is an associative array of arguments that can be passed in to configure the style:
- name: (required) a unique identifier, which is used to generate the CSS class (e.g.,
is-style-{name}
) - label: (required) an internationalized, human-readable label for the style
- inline_style: inline CSS to be printed when the style is in use
- style_handle: the handle of a registered stylesheet to enqueue for the style
- is_default: whether the style should be selected as the default for the block
To register a custom style, add a callback function in your theme’s functions.php
file and add it to the init
action hook. This code registers the hand-drawn
block style:
add_action( 'init', 'themeslug_register_block_styles' );
function themeslug_register_block_styles() {
register_block_style( 'core/image', array(
'name' => 'hand-drawn',
'label' => __( 'Hand-Drawn', 'themeslug' ),
'inline_style' => '.wp-block-image.is-style-hand-drawn img {
border: 2px solid currentColor;
overflow: hidden;
box-shadow: 0 4px 10px 0 rgba( 0, 0, 0, 0.3 );
border-radius: 255px 15px 225px 15px/15px 225px 15px 255px !important;
}'
) );
}
You can also register block styles via JavaScript.
How to unregister block styles?
add_action( 'init', 'themeslug_unregister_block_styles', 15 );
function themeslug_unregister_block_styles() {
unregister_block_style( 'core/image', 'hand-drawn' );
}
If the style was registered via JavaScript, it must also be unregistered via JavaScript.
Assuming a custom block-editor.js
file located in your theme’s assets/js
folder, enqueue the JavaScript as shown in the following code snippet:
add_action( 'enqueue_block_editor_assets', 'themeslug_block_editor_assets' );
function themeslug_block_editor_assets() {
wp_enqueue_script(
'themeslug-block-editor',
get_theme_file_uri( 'assets/js/block-editor.js' ),
array(
'wp-blocks',
'wp-dom-ready',
'wp-edit-post'
)
);
}
Add the following code to your block-editor.js
file to unregister the core rounded
style on the Image block:
wp.domReady( function () {
wp.blocks.unregisterBlockStyle( 'core/image', 'rounded' );
} );
Thanks for reading this article. This post is based on Creating custom block styles in WordPress themes
Leave a Reply