Let’s build a Gutenberg block that fetches posts via the REST API and displays them in a grid.
Gutenberg, the block editor in WordPress, offers developers immense flexibility to create custom blocks tailored to their needs. In this blog post, we’ll build a custom Gutenberg block that fetches and displays posts using the WordPress REST API in both the backend and frontend.
Why Use the REST API for a Gutenberg Block?
The WordPress REST API allows developers to interact with WordPress data seamlessly. By integrating it into a Gutenberg block, we can dynamically fetch and display posts without relying on hardcoded content.
Step 1: Create the Plugin File
We’ll create a single-file plugin for simplicity. Create a file named wp-rest-api-grid-block.php
in your wp-content/plugins
folder.
/*
Plugin Name: WP REST API Grid Block
Description: A Gutenberg block that fetches posts via the REST API and displays them in a grid.
Version: 1.0
Author: Your Name
*/
if (!defined('ABSPATH')) {
exit; // Exit if accessed directly.
}
Step 2: Register the Gutenberg Block
We’ll use register_block_type
to register the block and enqueue the necessary scripts.
function wp_rest_api_grid_block_assets() {
wp_register_script(
'wp-rest-api-grid-block',
false,
['wp-blocks', 'wp-element', 'wp-components', 'wp-api-fetch'],
null,
true
);
wp_add_inline_script(
'wp-rest-api-grid-block',
"(function(wp) {
const { registerBlockType } = wp.blocks;
const { useState, useEffect } = wp.element;
const { apiFetch } = wp;
registerBlockType('custom/wp-rest-api-grid-block', {
title: 'Posts Grid Block',
icon: 'grid-view',
category: 'widgets',
edit: () => {
const [posts, setPosts] = useState([]);
const [loading, setLoading] = useState(true);
useEffect(() => {
apiFetch({ path: '/wp/v2/posts' })
.then((response) => {
setPosts(response);
setLoading(false);
});
}, []);
if (loading) {
return 'Loading posts...';
}
return (
wp.element.createElement('div', { className: 'wp-rest-api-grid' },
wp.element.createElement('div', {
style: {
display: 'grid',
gridTemplateColumns: 'repeat(3, 1fr)',
gap: '16px'
}
},
posts.map(post => (
wp.element.createElement('div', {
key: post.id,
style: {
border: '1px solid #ccc',
padding: '10px'
}
},
wp.element.createElement('h3', {
dangerouslySetInnerHTML: { __html: post.title.rendered }
}),
wp.element.createElement('p', {
dangerouslySetInnerHTML: { __html: post.excerpt.rendered }
})
)
))
)
)
);
},
save: () => {
return null;
},
});
})(window.wp);"
);
register_block_type('custom/wp-rest-api-grid-block', array(
'editor_script' => 'wp-rest-api-grid-block',
'render_callback' => 'wp_rest_api_grid_block_render'
));
}
add_action('init', 'wp_rest_api_grid_block_assets');
Step 3: Add Server-Side Rendering for Frontend
In the frontend, we’ll dynamically render the posts using a render_callback
.
function wp_rest_api_grid_block_render() {
$posts = get_posts([
'numberposts' => 6,
'post_status' => 'publish',
]);
if (empty($posts)) {
return '<p>No posts found.</p>';
}
$output = '<div class="wp-rest-api-grid" style="display: grid; grid-template-columns: repeat(3, 1fr); gap: 16px;">';
foreach ($posts as $post) {
$output .= '<div style="border: 1px solid #ccc; padding: 10px;">';
$output .= '<h3>' . esc_html($post->post_title) . '</h3>';
$output .= '<p>' . esc_html(wp_trim_words($post->post_content, 20)) . '</p>';
$output .= '</div>';
}
$output .= '</div>';
return $output;
}
Step 4: Activate the Plugin
- Go to Plugins > Installed Plugins.
- Activate “WP REST API Grid Block”.
Step 5: Add the Block to a Page or Post
- Open the Block Editor.
- Search for “Posts Grid Block”.
- Add it to your content.
- Publish the post/page.
Frontend Preview
You should now see a beautiful grid of recent posts displayed dynamically.
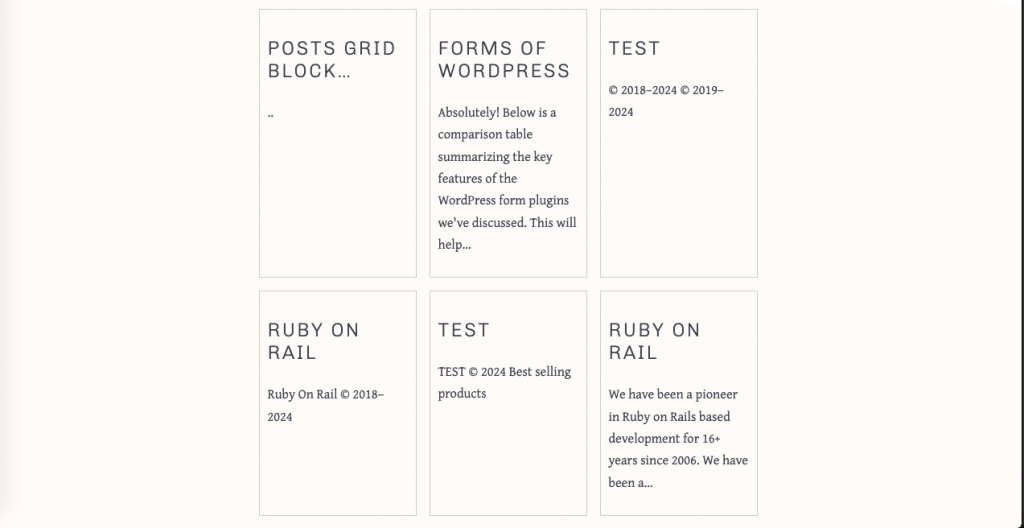
Conclusion
Congratulations! 🎉 You’ve successfully built a custom Gutenberg block that uses the WordPress REST API to display posts dynamically in a grid. This approach showcases the power of both Gutenberg blocks and the REST API for building dynamic and engaging WordPress experiences.
If you encounter any issues or have questions, feel free to drop a comment below!
Here you can find the complete code.
Leave a Reply