If you have purchased anything on Amazon, you might have seen a section called Customer Questions & Answers near the rating & review section. You can find questions & their answers if you search there. These customer’s questions are answered by the Merchant or by the other users.
We are going to build a similar feature for WooCommerce products. On the product single page (PDP), we’ll add another tab called Questions & Answers.
Let’s add some code snippets
/**
* Add a Questions & Answers tab
*/
add_filter( 'woocommerce_product_tabs', 'wc_questions_answers_tab' );
function wc_questions_answers_tab( $tabs ) {
// Adds the new tab
$tabs['questions_answers_tab'] = array(
'title' => __( 'Customers Questions & Answers', 'woocommerce' ),
'priority' => 50,
'callback' => 'wc_questions_answers_tab_content'
);
return $tabs;
}
function wc_questions_answers_tab_content() {
echo '<h2>Customers Questions And Answers</h2>';
echo '<p>Here\'s your Questions And Answers tab.</p>';
}
It’ll add a new section next to the Reviews Tab.
But we want to give an option where customers can ask Questions. Also, we need to display all the questions & answers.
We can use the comment feature of WordPress as a question box. There is a function called comment_form() to show a comment form. Also, we’ll use wp_list_comments to display all the discussions.
add_filter( 'woocommerce_product_tabs', 'wc_questions_answers_tab' );
function wc_questions_answers_tab( $tabs ) {
// Adds the new tab
$tabs['questions_answers_tab'] = array(
'title' => __( 'Customers Questions & Answers', 'woocommerce' ),
'priority' => 50,
'callback' => 'wc_questions_answers_tab_content'
);
return $tabs;
}
function wc_questions_answers_tab_content() {
?>
<div id="discussion" class="woocommerce-discussion">
<div id="discussion-list">
<ol class="commentlist">
<?php wp_list_comments(); ?>
</ol>
</div>
<div id="question-form">
<?php
comment_form();
?>
</div>
</div>
<?php
}
Now the code looks like this. It will create an odd-looking section like below. You can ignore the layout for the time being.
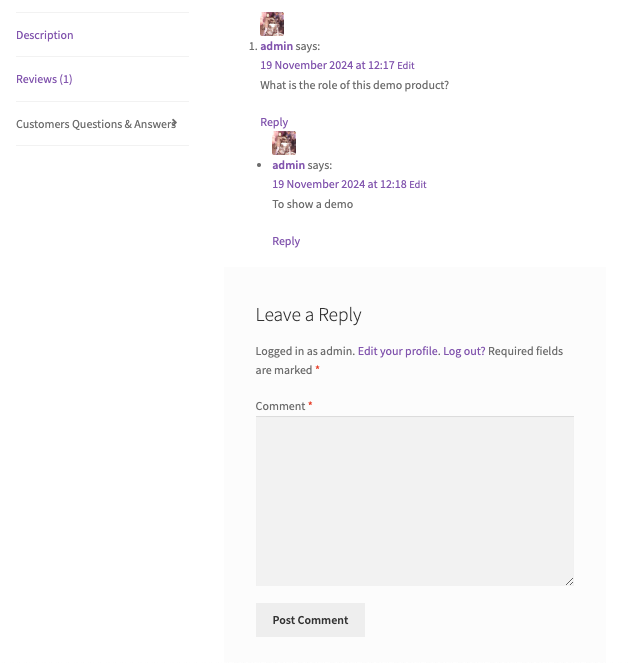
Now we need to refactor the code so we can build a more useable plugin. After a few changes & code refactoring, I’m able to show comments in Tabs & now each Question will be opening on the new page under that product where admin/customers can answer that Question.
Here is the plugin added to GitHub.
Let me explain a few codes from this plugin.
// Modify the comment link to point to the new page for answers under product pages
function modify_comment_link( $link, $comment ) {
// Ensure it's a parent comment of type 'discussion'
if ( $comment->comment_parent == 0 && $comment->comment_type == 'discussion' ) {
// Get the product associated with the comment
$product = get_post( $comment->comment_post_ID );
if ( $product && 'product' === $product->post_type ) {
// Generate the link for the discussion page under the product
$link = home_url( '/' . $product->post_name . '/discussion/' . $comment->comment_ID . '/' );
}
}
return $link;
}
add_filter( 'get_comment_link', 'modify_comment_link', 10, 2 );
On the Customers Q&As Tab, we are adding a link to Question (Parent Comment) a link. So now the comment would open on a new page with a link like yourstore.com/{product-name}/discussion/{comment_id}
Let’s add some URL rewrite rules.
// Register custom rewrite rules for the comment answers page under the product
function comment_answers_rewrite_rule() {
// Add a custom rewrite rule: /product-name/discussion/comment-id
add_rewrite_rule(
'^([^/]+)/discussion/([0-9]+)/?', // URL structure: product-name/discussion/comment-id
'index.php?product_slug=$matches[1]&comment_answers_id=$matches[2]', // Map to query vars
'top'
);
}
add_action( 'init', 'comment_answers_rewrite_rule', 999 );
// Add custom query vars for product slug and comment answers ID
function add_comment_answers_query_vars( $query_vars ) {
$query_vars[] = 'product_slug'; // Add 'product_slug' query var
$query_vars[] = 'comment_answers_id'; // Add 'comment_answers_id' query var
return $query_vars;
}
add_filter( 'query_vars', 'add_comment_answers_query_vars' );
You can dig deeper into the plugin & explore the code.
Here are the final outcomes.
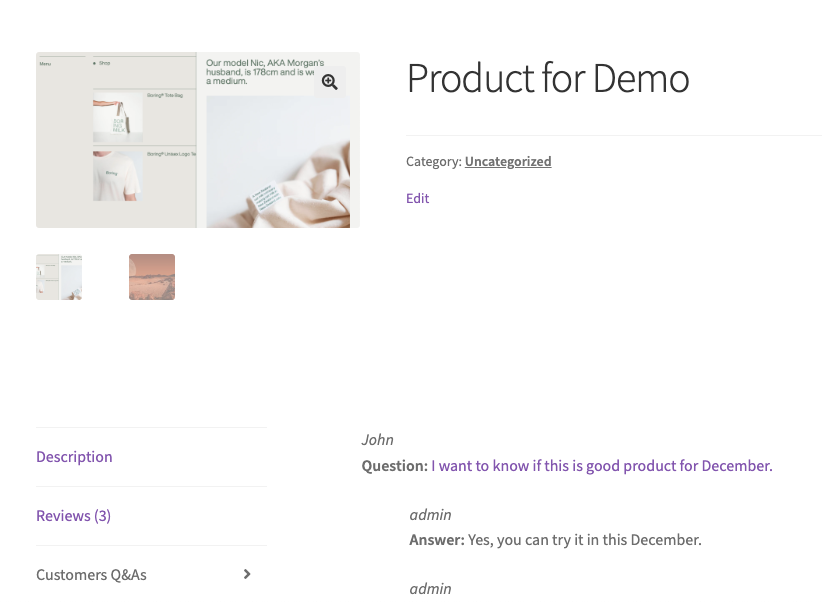
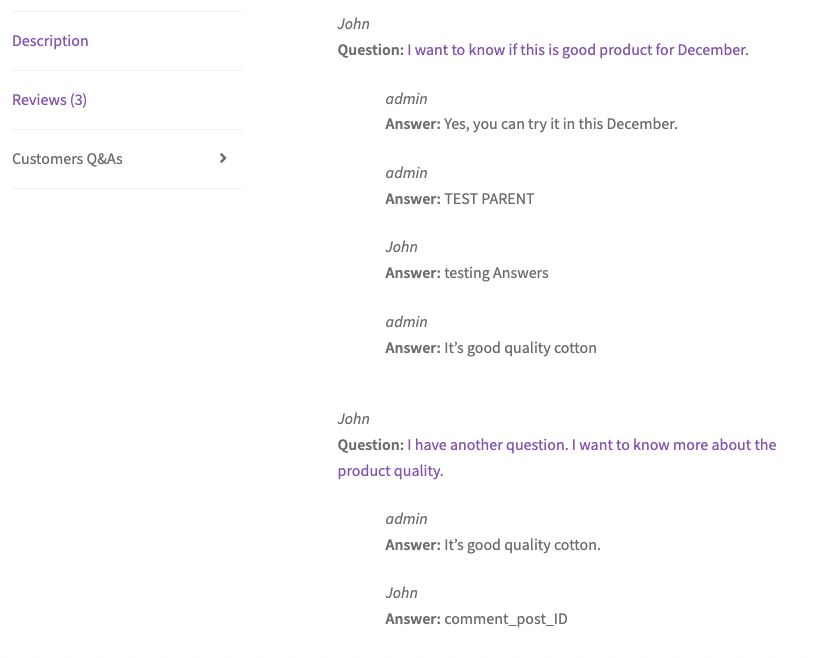
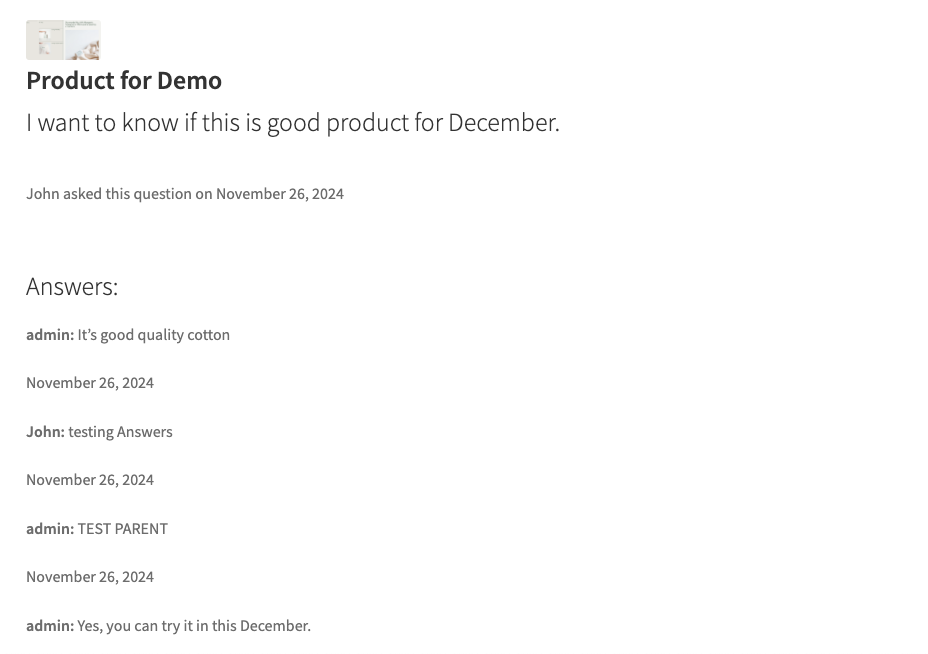
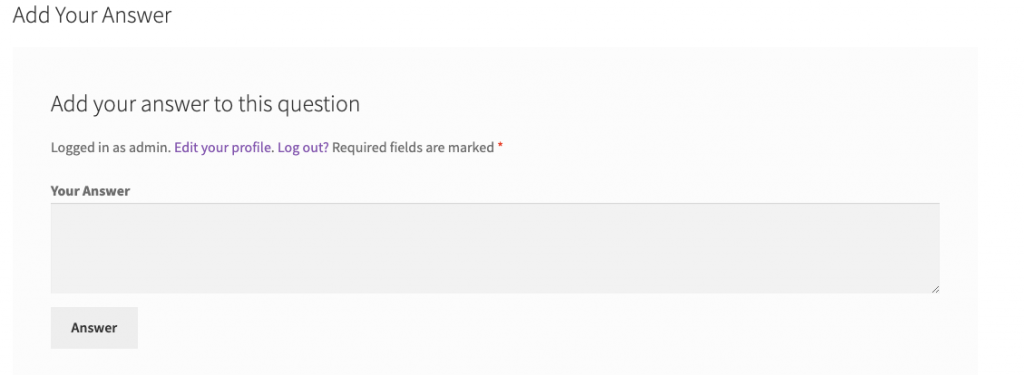
So this plugin would be adding a new tab on WooCommerce Store PDP for Q&As. Now, customers can ask Questions on the Product page. It can be answered by the admin or store manager in the Reviews section of wp-admin or by going to the question page in the front-end.
Adding Questions to your eCommerce store is a good way to clear doubts & do SEO.
Leave a Reply