Let’s Learn How to Add a File Upload Field to WooCommerce Checkout Without a Plugin.
Adding a file upload field to the WooCommerce checkout page is a powerful customization for stores selling personalized or custom products—like print-on-demand, engraving, or document processing. In this guide, you’ll learn how to add, validate, and save a file upload field using code that works in both classic and block-based WordPress themes.
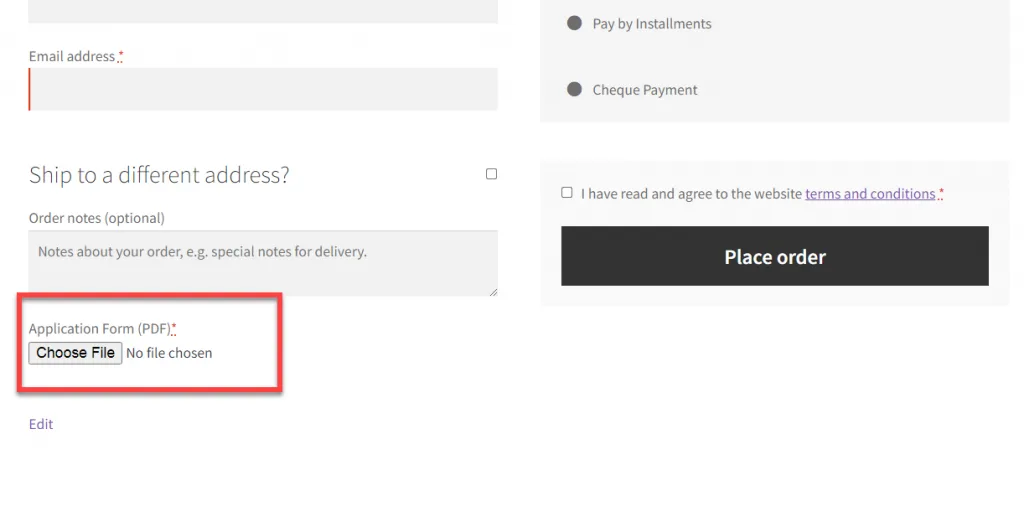
Use Case Scenarios
- Print shops accepting artwork
- Custom gift sellers accepting design files
- Legal/financial firms requiring ID uploads
- Tailor shops accepting measurements or specifications
Step-by-Step Code Tutorial
Important: Add all code snippets to your theme’s
functions.php
file or a site-specific plugin. Use a child theme to avoid losing changes on updates.
1. Add the File Upload Field to Checkout
This code adds a file input below the order notes section:
add_action( 'woocommerce_after_order_notes', 'add_custom_checkout_file_upload' );
function add_custom_checkout_file_upload( $checkout ) {
echo '<div class="woocommerce-additional-fields__field-wrapper">';
woocommerce_form_field( 'upload_file', array(
'type' => 'file',
'class' => array( 'form-row-wide' ),
'label' => __( 'Upload Your File', 'woocommerce' ),
'required' => true,
'accept' => '.jpg,.jpeg,.png,.pdf',
), $checkout->get_value( 'upload_file' ) );
echo '</div>';
}
2. Validate the Upload on Checkout Submit
This ensures the user has uploaded a file:
add_action( 'woocommerce_checkout_process', 'validate_file_upload' );
function validate_file_upload() {
if ( empty( $_FILES['upload_file'] ) || $_FILES['upload_file']['error'] !== UPLOAD_ERR_OK ) {
wc_add_notice( __( 'Please upload a file before placing your order.', 'woocommerce' ), 'error' );
}
}
3. Save the File with the Order
Move the uploaded file to the WordPress uploads folder and save the file path with the order:
add_action( 'woocommerce_checkout_create_order', 'save_uploaded_file_to_order', 10, 2 );
function save_uploaded_file_to_order( $order, $data ) {
if ( isset( $_FILES['upload_file'] ) && $_FILES['upload_file']['error'] === UPLOAD_ERR_OK ) {
$upload_dir = wp_upload_dir();
$file_name = basename( $_FILES['upload_file']['name'] );
$target_path = $upload_dir['path'] . '/' . $file_name;
if ( move_uploaded_file( $_FILES['upload_file']['tmp_name'], $target_path ) ) {
$order->update_meta_data( '_uploaded_file_url', $upload_dir['url'] . '/' . $file_name );
}
}
}
4. Show the Uploaded File in the Admin Panel
Display the file link in the order admin screen:
add_action( 'woocommerce_admin_order_data_after_billing_address', 'display_uploaded_file_in_admin_order', 10, 1 );
function display_uploaded_file_in_admin_order( $order ) {
$file_url = $order->get_meta( '_uploaded_file_url' );
if ( $file_url ) {
echo '<p><strong>' . __( 'Uploaded File', 'woocommerce' ) . ':</strong> <a href="' . esc_url( $file_url ) . '" target="_blank">' . basename( $file_url ) . '</a></p>';
}
}
Security and Best Practices
- Restrict file types: Only allow safe formats (.jpg, .png, .pdf, etc.)
- Limit file size: Add server-side checks if necessary.
- Sanitize file names: Prevent directory traversal or injection attacks.
- Use non-public folders if you need to keep uploaded files private.
Plugin Alternative
If you prefer not to code, try plugins like:
- Checkout Files Upload for WooCommerce
- WooCommerce Checkout Manager Pro
Leave a Reply