Gutenberg, the block editor in WordPress, allows developers to create powerful custom blocks for dynamic content. In this blog post, we’ll build a custom Gutenberg block that fetches and displays weather information from an external Weather API in the WordPress editor.
Why Use an External API in a Gutenberg Block?
Using external APIs in Gutenberg blocks allows you to pull in dynamic data, such as weather updates, stock prices, or social media feeds, directly into your WordPress posts or pages.
Looking for WooCommerce Development?
Step 1: Create the Plugin File
Create a file named wp-weather-api-block.php
in your wp-content/plugins
folder.
/*
Plugin Name: WP Weather API Block
Description: A Gutenberg block that fetches weather data from an external API and displays it.
Version: 1.0
Author: Kishore
*/
if (!defined('ABSPATH')) {
exit; // Exit if accessed directly.
}
Step 2: Register the Gutenberg Block
We’ll use register_block_type
to set up the block and enqueue the necessary scripts.
function wp_weather_api_block_assets() {
wp_register_script(
'wp-weather-api-block',
false,
['wp-blocks', 'wp-element', 'wp-components', 'wp-api-fetch'],
null,
true
);
wp_add_inline_script(
'wp-weather-api-block',
"(function(wp) {
const { registerBlockType } = wp.blocks;
const { useState, useEffect } = wp.element;
registerBlockType('custom/wp-weather-api-block', {
title: 'Weather API Block',
icon: 'cloud',
category: 'widgets',
edit: () => {
const [weather, setWeather] = useState(null);
const [loading, setLoading] = useState(true);
useEffect(() => {
fetch('https://api.openweathermap.org/data/2.5/weather?q=London&appid=YOUR_API_KEY')
.then(response => response.json())
.then(data => {
if (data.cod === 401) {
setWeather({ error: 'Invalid API Key. Please check your configuration.' });
} else {
setWeather(data);
}
setLoading(false);
})
.catch(error => {
console.error('Error:', error);
setLoading(false);
});
}, []);
if (loading) {
return 'Loading weather data...';
}
if (weather && weather.error) {
return weather.error;
}
if (!weather) {
return 'Failed to load weather data.';
}
return (
wp.element.createElement('div', { className: 'wp-weather-api-block' },
wp.element.createElement('h3', null, 'Weather in ' + weather.name),
wp.element.createElement('p', null, 'Temperature: ' + (weather.main.temp - 273.15).toFixed(2) + '°C'),
wp.element.createElement('p', null, 'Weather: ' + weather.weather[0].description)
)
);
},
save: () => {
return null;
},
});
})(window.wp);"
);
register_block_type('custom/wp-weather-api-block', array(
'editor_script' => 'wp-weather-api-block',
'render_callback' => 'wp_weather_api_block_render'
));
}
add_action('init', 'wp_weather_api_block_assets');
Step 3: Server-Side Rendering for Frontend
For frontend display, we’ll fetch weather data dynamically.
function wp_weather_api_block_render() {
$api_key = 'YOUR_API_KEY';
$city = 'London';
$response = wp_remote_get("https://api.openweathermap.org/data/2.5/weather?q={$city}&appid={$api_key}");
if (is_wp_error($response)) {
return '<p>Failed to fetch weather data.</p>';
}
$weather_data = json_decode(wp_remote_retrieve_body($response), true);
if (!$weather_data || isset($weather_data['cod']) && $weather_data['cod'] != 200) {
$error_message = isset($weather_data['message']) ? $weather_data['message'] : 'Invalid weather data received.';
return "<p>Error: {$error_message}</p>";
}
$temp = round($weather_data['main']['temp'] - 273.15, 2);
$description = $weather_data['weather'][0]['description'];
$city_name = $weather_data['name'];
return "<div class='wp-weather-api-block'>
<h3>Weather in {$city_name}</h3>
<p>Temperature: {$temp}°C</p>
<p>Weather: {$description}</p>
</div>";
}
Step 4: Activate the Plugin
- Go to Plugins > Installed Plugins.
- Activate “WP Weather API Block”.
Step 5: Add the Block to a Page or Post
- Open the Block Editor.
- Search for “Weather API Block”.
- Add it to your content.
- Publish the post/page.
Frontend Preview
You should now see real-time weather data displayed in your block.
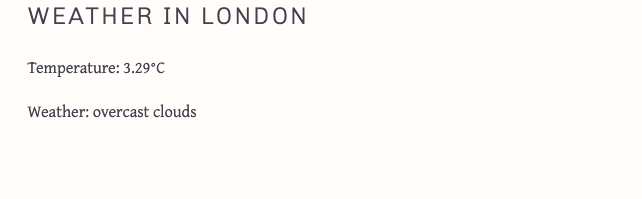
Conclusion
You’ve successfully created a Gutenberg block that fetches real-time weather data from an external Weather API and displays it dynamically in both the backend editor and frontend view.
Make sure to replace YOUR_API_KEY
with your actual API key from OpenWeatherMap.
If you encounter any issues or have questions, feel free to drop a comment below!
Leave a Reply